Create the form as on the picture bellow!
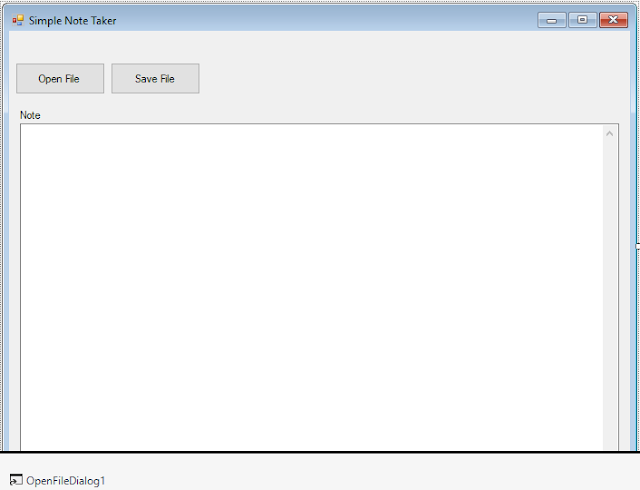
Controls properties are following:
TextBox1: .Name = txtNote .ScrollBars = Vertical
Button1: .Name = btnOpen
Button2: .Name = btnSave
Open Text Files in Visual Basic 2015 TextBox Control
To be able to open files in TextBox control we have to add OpenFile Dialog at first. In the picture bellow you see how to add it: Toolbox - OpenFileDialog. You just click on OpenFileDialog and go in the form - somewhere, left click on it and OpenFileDialog will be inserted automatically.
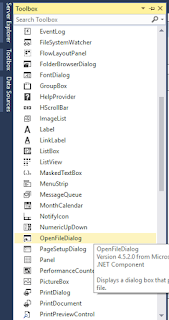
Here is the code for opening Text Files in TextBox control.
Private Sub btnOpen_Click(sender As Object, e As EventArgs) Handles btnOpen.Click
Dim oReader As StreamReader
OpenFileDialog1.CheckFileExists = True
OpenFileDialog1.CheckPathExists = True
OpenFileDialog1.DefaultExt = "txt"
OpenFileDialog1.FileName = ""
OpenFileDialog1.Filter = "Text Files (*.txt)|*.txt|All Files (*.*)|*.*"
OpenFileDialog1.Multiselect = False
If OpenFileDialog1.ShowDialog = DialogResult.OK Then
oReader = New StreamReader(OpenFileDialog1.FileName, True)
txtNote.Text = oReader.ReadToEnd
End If
End Sub
Save Text File from TextBox in Visual Basic 2015
First add SaveFileDialog in the form to be able to open Save Dialog and save the content from the textbox.
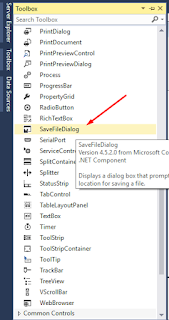
Here is the code to save the content from TextBox in Text File. I also added an option to save in other extension if you wish.
Private Sub btnSave_Click(sender As Object, e As EventArgs) Handles btnSave.Click
SaveFileDialog1.DefaultExt = "txt"
SaveFileDialog1.FileName = ""
SaveFileDialog1.Filter = "Text Files (*.txt)|*.txt|All Files (*.*)|*.*"
SaveFileDialog1.FilterIndex = 2
SaveFileDialog1.RestoreDirectory = True
If SaveFileDialog1.ShowDialog() = DialogResult.OK Then
IO.File.WriteAllText(SaveFileDialog1.FileName, txtNote.Text)
End If
End Sub
great. thank you. exactly what I needed
ReplyDeletethank you. I try to share as much as possible
ReplyDelete